The latest Raspberry Pi 4 comes with an option of 4G ram. This truly makes the Raspberry Pi a workable personal portable computer. However, the temperature could go pretty high when it is implementing intensive tasks. The simplest solution would be adding a fan to cool it down, but it makes no sense to let the fan running all the time.
In this tutorial, we are going to build a auto start/stop 5V Fan for the Raspberry Pi 4 based on the temperature of CPU.
Preparation
You might need the following things other than a Raspberry Pi:
- a
5V Fan
, ideally with a case that has the slots for the fan; - a
transistor
to work as a electronic switch, I used NPN transistor; - a
1kΩ
resistor to protect the transistor from overheat; - a
breadboard
for prototyping (not necessary, but recommended) - few jumper wires (mainly male to male, male to female)
Wire Up
Notice the 3 pins for the transistor from left to right are E, B, C. Make sure the E is connected to the positive end (anode), B to the GPIO through a 1kΩ resistor, C to the GND of the Raspberry Pi.
Here is a good explanation of a transistor's pins: NPN 2N222 Transistor...with schematic tutorial
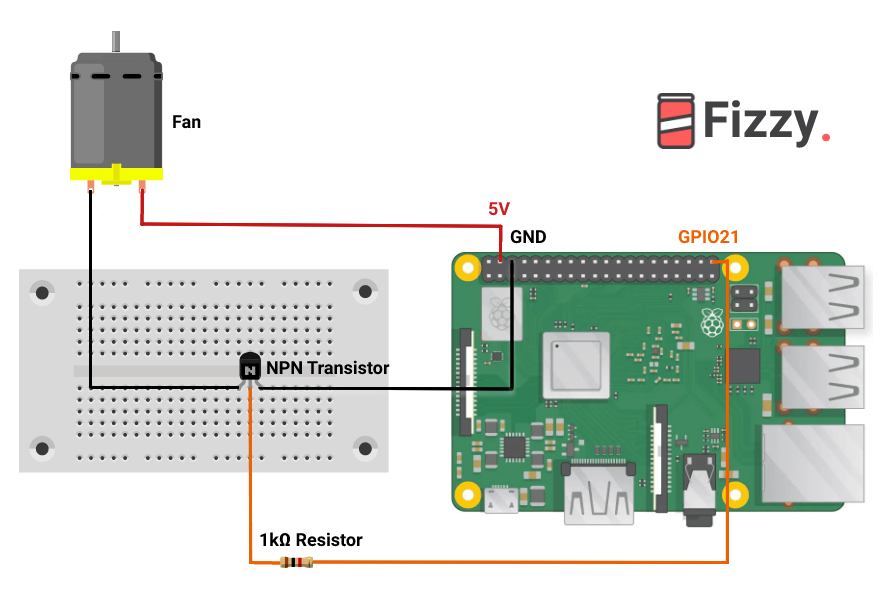
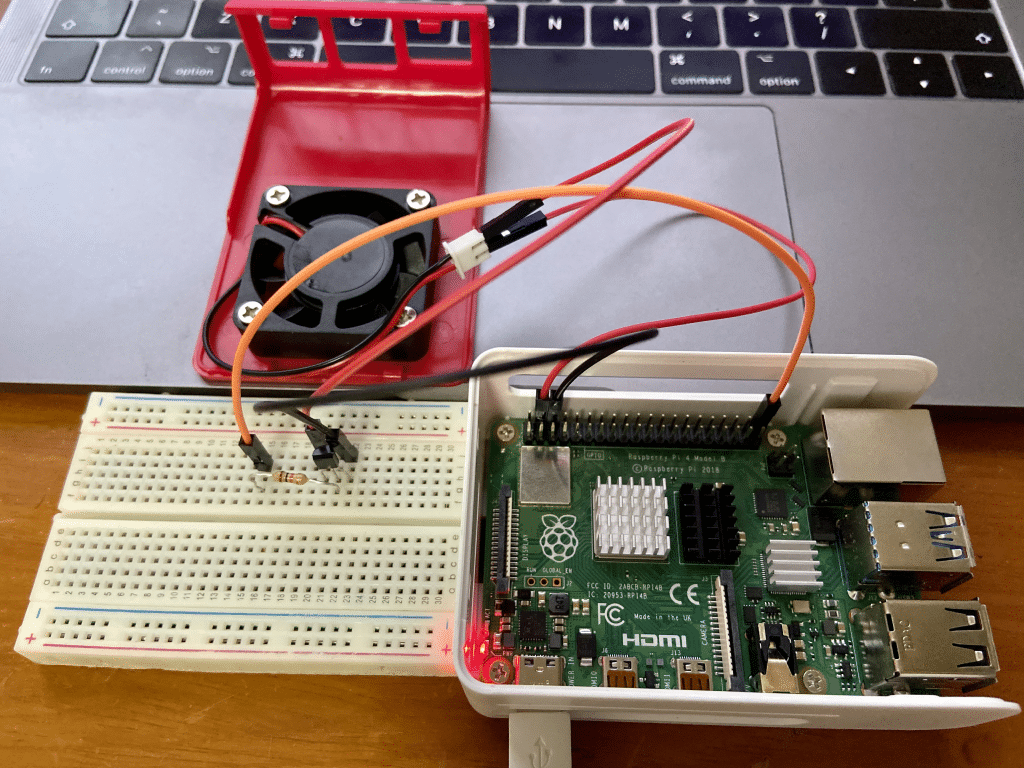
Code
We use Python to control the GPIO, and set the script to run after the Raspberry Pi system boot.
First, create a Python file in /home/pi/Scripts/
, name as fan.py
. We simply use the code for blink a LED from gpiozero
package to control the GPIO, documentation: https://gpiozero.readthedocs.io/en/stable/index.html.
If you didn't wire the jumper wires as I did, modify the GPIO accordingly. GPIO reference: Notes for Raspberry Pi
We can test it by execute python /home/pi/Scripts/fan.py
, you can see the log in the terminal. Now the script is working, it will monitor the temperature of the CPU, then turn on/off the fan base on the threshold degrees we set. The only problem is we have to execute the command to run it every time we reboot the system.
You can create a bash alias for this execution command, but here I'm going to show you how to add this script as a service to your Raspberry Pi and make it running after every time we reboot.
Create a service file under /etc/init.d
called fan
, so the file path looks like /etc/init.d/fan
, edit the file and paste the following:
Then we make it executable:
Use the following command to control the fan service:
Make it running after reboot:
We can test whether it is added to the list:
Finally we need to reboot the Raspberry Pi to make the changes effective:
Further Improvements
After finish the testing, you can solder things up and put them into the case to make it prettier. Moreover, you could try to use GPIO to output PMW to control the speed of the fan, ref: https://blog.csdn.net/r0ck_y0u/article/details/89498804.